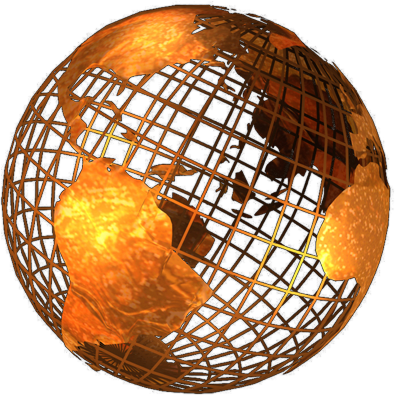
In reactive design, the aim to is make a website design fit within almost any size of screen. We can specifically decide how the page should look, depending on the available space. Consider this:
@media screen and (min-width: 1px) {
body {width: 732px;}
}
@media screen and (min-width: 972px) {
body {width: 952px;}
}
@media screen and (min-width: 1192px) {
body {width: 1172px;}
}
For each size of screen, we have decided to make the body a specific width, to ensure we have a "body" which fits the screen. The exception to this is very small screens, which we show the narrowest possible width.
If, within our design we have div's which flow across the screen...
div.someThing {width XXpx; float: left; margin-right: 10px;}
...we can calculate how many will fit. This calculation must however take into account the entire width of the div, including any border, padding or margin.
Here comes the interesting part... We can strip the margin from the right side of a div, based on nth-child.
So lets say we can fit 2, 3 or 4 boxes across on the design above. It seems obvious that...
@media screen and (min-width: 1px) {
body {width: 732px;}
div.someThing:nth-child(2n+2) {margin-right: 0px;}
}
@media screen and (min-width: 972px) {
body {width: 952px;}
div.someThing:nth-child(3n+3) {margin-right: 0px;}
}
@media screen and (min-width: 1192px) {
body {width: 1172px;}
div.someThing:nth-child(4n+4) {margin-right: 0px;}
}
...would work. This looks like it would indeed strip the right margin from the right of the boxes.
This does not work however because the first "C" of CSS is "Cascading". To put that more clearly, for a screen with of 1280px, all three of the @media rules above apply, so the 2nd, 3rd and 4th elements are all matched. So the 2nd, 3rd and 4th div's are stripped of their right margin.
When we declare margin-right for the nth-child div's, we need to re-set the previous versions:
@media screen and (min-width: 1px) {
body {width: 732px;}
div.someThing:nth-child(2n+2) {margin-right: 0px;}
}
@media screen and (min-width: 972px) {
body {width: 952px;}
div.someThing:nth-child(2n+2) {margin-right: 10px;}
div.someThing:nth-child(3n+3) {margin-right: 0px;}
}
@media screen and (min-width: 1192px) {
body {width: 1172px;}
div.someThing:nth-child(2n+2) {margin-right: 10px;}
div.someThing:nth-child(3n+3) {margin-right: 10px;}
div.someThing:nth-child(4n+4) {margin-right: 0px;}
}
Ensuring that the previous @media declarations are over-written will keep the design flowing as expected.
Clive Knowles 2014-08-05